tenbulls blog
...attaining enlightenment with the Microsoft Data and Cloud Platforms with a sprinkling of Open Source and related technologies!
Welcome to the Tenbulls Blog! In these pages I share some of my technology insights with the Global IT Community and sometimes ramble about my professional experiences. Hope you find some of this useful.
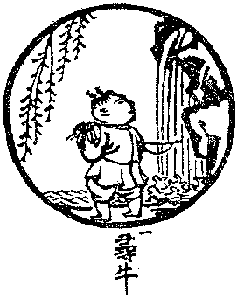
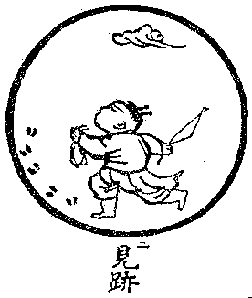
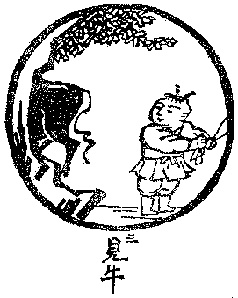
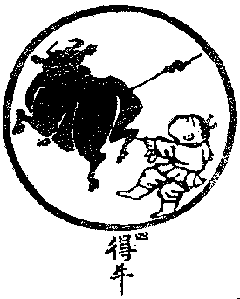
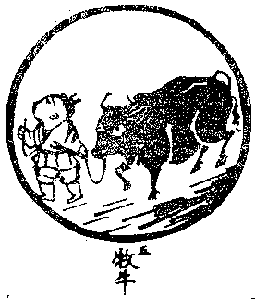
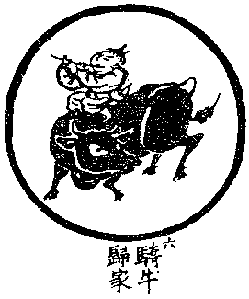
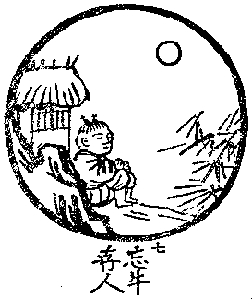
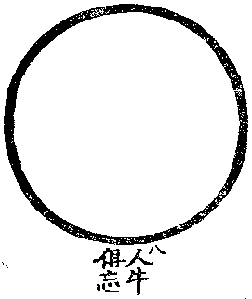
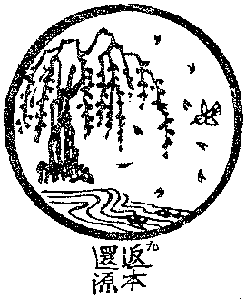
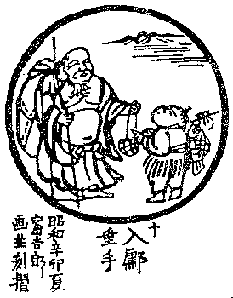